mirror of
https://github.com/cargo-bins/cargo-binstall.git
synced 2025-07-01 15:06:37 +00:00
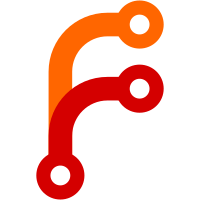
* Rm unnecessary warning msg "Failed to read git credential file" Fixed #1476 If `gh auth token` executed successfully and binstall obtained a gh token from it, then there's no reason to issue any warning msg. Only when binstall cannot read from `.git-credential` and `gh auth token` failed does binstall need to issue warning. Signed-off-by: Jiahao XU <Jiahao_XU@outlook.com> * Fix clippy warning Signed-off-by: Jiahao XU <Jiahao_XU@outlook.com> --------- Signed-off-by: Jiahao XU <Jiahao_XU@outlook.com>
66 lines
1.6 KiB
Rust
66 lines
1.6 KiB
Rust
use std::{env, fs, path::PathBuf};
|
|
|
|
use compact_str::CompactString;
|
|
use dirs::home_dir;
|
|
|
|
pub fn try_from_home() -> Option<CompactString> {
|
|
if let Some(mut home) = home_dir() {
|
|
home.push(".git-credentials");
|
|
if let Some(cred) = from_file(home) {
|
|
return Some(cred);
|
|
}
|
|
}
|
|
|
|
if let Some(home) = env::var_os("XDG_CONFIG_HOME") {
|
|
let mut home = PathBuf::from(home);
|
|
home.push("git/credentials");
|
|
|
|
if let Some(cred) = from_file(home) {
|
|
return Some(cred);
|
|
}
|
|
}
|
|
|
|
None
|
|
}
|
|
|
|
fn from_file(path: PathBuf) -> Option<CompactString> {
|
|
fs::read_to_string(path)
|
|
.ok()?
|
|
.lines()
|
|
.find_map(from_line)
|
|
.map(CompactString::from)
|
|
}
|
|
|
|
fn from_line(line: &str) -> Option<&str> {
|
|
let cred = line
|
|
.trim()
|
|
.strip_prefix("https://")?
|
|
.strip_suffix("@github.com")?;
|
|
|
|
Some(cred.split_once(':')?.1)
|
|
}
|
|
|
|
#[cfg(test)]
|
|
mod test {
|
|
use super::*;
|
|
|
|
const GIT_CREDENTIALS_TEST_CASES: &[(&str, Option<&str>)] = &[
|
|
// Success
|
|
("https://NobodyXu:gho_asdc@github.com", Some("gho_asdc")),
|
|
(
|
|
"https://NobodyXu:gho_asdc12dz@github.com",
|
|
Some("gho_asdc12dz"),
|
|
),
|
|
// Failure
|
|
("http://NobodyXu:gho_asdc@github.com", None),
|
|
("https://NobodyXu:gho_asdc@gitlab.com", None),
|
|
("https://NobodyXugho_asdc@github.com", None),
|
|
];
|
|
|
|
#[test]
|
|
fn test_extract_from_line() {
|
|
GIT_CREDENTIALS_TEST_CASES.iter().for_each(|(line, res)| {
|
|
assert_eq!(from_line(line), *res);
|
|
})
|
|
}
|
|
}
|