mirror of
https://github.com/cargo-bins/cargo-binstall.git
synced 2025-04-22 21:48:42 +00:00
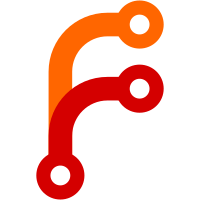
The startup/init code in `entry::install_crates` performs a lot of blocking operations, from testing if dir exists to reading from files and there is no `.await` in there until after the startup. There are also a few cases where `block_in_place` should be called (e.g. loading manifests, loading TLS certificates) but is missing. Most of the `Args` passed to `entry::install_crates` are actually consumed before the first `.await` point, so performing startup/init code eagerly would make the generated future much smaller, reduce codegen and also makes it easier to optimize. Signed-off-by: Jiahao XU <Jiahao_XU@outlook.com>
42 lines
987 B
Rust
42 lines
987 B
Rust
use std::time::Instant;
|
|
|
|
use binstalk::helpers::jobserver_client::LazyJobserverClient;
|
|
use log::LevelFilter;
|
|
use tracing::debug;
|
|
|
|
use cargo_binstall::{
|
|
args,
|
|
bin_util::{run_tokio_main, MainExit},
|
|
entry,
|
|
logging::logging,
|
|
};
|
|
|
|
#[cfg(feature = "mimalloc")]
|
|
#[global_allocator]
|
|
static GLOBAL: mimalloc::MiMalloc = mimalloc::MiMalloc;
|
|
|
|
fn main() -> MainExit {
|
|
// This must be the very first thing to happen
|
|
let jobserver_client = LazyJobserverClient::new();
|
|
|
|
let args = args::parse();
|
|
|
|
if args.version {
|
|
println!("{}", env!("CARGO_PKG_VERSION"));
|
|
MainExit::Success(None)
|
|
} else {
|
|
logging(
|
|
args.log_level.unwrap_or(LevelFilter::Info),
|
|
args.json_output,
|
|
);
|
|
|
|
let start = Instant::now();
|
|
|
|
let result = run_tokio_main(|| entry::install_crates(args, jobserver_client));
|
|
|
|
let done = start.elapsed();
|
|
debug!("run time: {done:?}");
|
|
|
|
MainExit::new(result, done)
|
|
}
|
|
}
|