mirror of
https://github.com/cargo-bins/cargo-binstall.git
synced 2025-04-22 13:38:43 +00:00
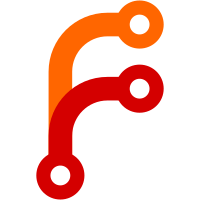
- Refactor: Mv fn `utils::asyncify` into mod `utils` - Improve err msg for task failure in `utils::asyncify` - Make sure `asyncify` always returns the same annoymous type that implements `Future` if the `T` is same. - Rewrite `extract_bin` to avoid `block_in_place` support cancellation by dropping - Rm unused dep scopeguard from binstalk-downloader - Rewrite `extract_tar_based_stream` so that it is cancellable by dropping - Unbox `extract_future` in `async_extracter::extract_zip` - Refactor `Download` API: Remove `CancellationFuture` as param since all futures returned by `Download::and_*` does not call `block_in_place`, so they can be cancelled by drop instead of using this cumbersome hack. - Fix exports from mod `async_tar_visitor` - Make `signal::{ignore_signals, wait_on_cancellation_signal}` private - Rm the global variable `CANCELLED` in `wait_on_cancellation_signal` and rm fn `wait_on_cancellation_signal_inner` - Optimize `wait_on_cancellation_signal`: Avoid `tokio::select!` on `not(unix)` - Rm unnecessary `tokio::select!` in `wait_on_cancellation_signal` on unix Since `unix::wait_on_cancellation_signal_unix` already waits for ctrl + c signal. - Optimize `extract_bin`: Send `Bytes` to blocking thread for zero-copy - Optimize `extract_with_blocking_decoder`: Avoid dup monomorphization - Box fut of `fetch_crate_cratesio` in `PackageInfo::resolve` - Optimize `extract_zip_entry`: Spawn only one blocking task per fn call by using a mspc queue for the data to be written to the `outfile`. This would improve efficiency as using `tokio::fs::File` is expensive: It spawns a new blocking task, which needs one heap allocation and then pushed to a mpmc queue, and then wait for it to be done on every loop. This also fix a race condition where the unix permission is set before the whole file is written, which might be used by attackers. - Optimize `extract_zip`: Use one `BytesMut` for entire extraction process To avoid frequent allocation and deallocation. - Optimize `extract_zip_entry`: Inc prob of reusing alloc in `BytesMut` Performs the reserve before sending the buf over mpsc queue to increase the possibility of reusing the previous allocation. NOTE: `BytesMut` only reuses the previous allocation if it is the only one holds the reference to it, which is either on the first allocation or all the `Bytes` in the mpsc queue has been consumed, written to the file and dropped. Since reading from entry would have to wait for external file I/O, this would give the blocking thread some time to flush `Bytes` out. - Disable unused feature fs of dep tokio Signed-off-by: Jiahao XU <Jiahao_XU@outlook.com>
22 lines
675 B
Rust
22 lines
675 B
Rust
use std::{future::Future, io};
|
|
|
|
use tokio::task;
|
|
|
|
/// Copied from tokio https://docs.rs/tokio/latest/src/tokio/fs/mod.rs.html#132
|
|
pub(super) fn asyncify<F, T>(f: F) -> impl Future<Output = io::Result<T>> + Send + Sync + 'static
|
|
where
|
|
F: FnOnce() -> io::Result<T> + Send + 'static,
|
|
T: Send + 'static,
|
|
{
|
|
async fn inner<T: Send + 'static>(handle: task::JoinHandle<io::Result<T>>) -> io::Result<T> {
|
|
match handle.await {
|
|
Ok(res) => res,
|
|
Err(err) => Err(io::Error::new(
|
|
io::ErrorKind::Other,
|
|
format!("background task failed: {err}"),
|
|
)),
|
|
}
|
|
}
|
|
|
|
inner(task::spawn_blocking(f))
|
|
}
|